[Flutter] 메모 9
2020. 12. 28. 00:25ㆍFlutter Mobile App/Flutter 메모 앱
반응형
메모 삭제 기능
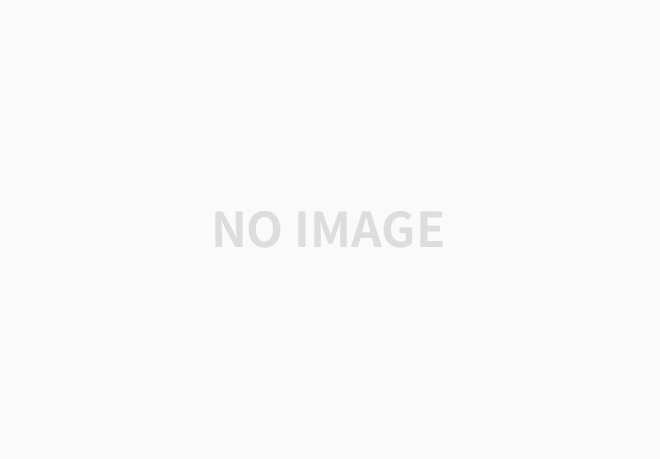
홈 페이지
[screens/home_page.dart]
더보기
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:memo/model/memo.dart';
import 'write_page.dart';
import 'package:memo/repository/db_helper.dart';
class HomePage extends StatefulWidget {
final String title;
HomePage({Key key, this.title}) : super(key: key);
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
String deleteId = '';
Future<String> _calculation = Future<String>.delayed(
Duration(seconds: 2),
() => 'Data Loaded',
);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
children: <Widget>[
Padding(
padding: EdgeInsets.only(left: 20, top: 40, bottom: 20),
child: Container(
child: Text(
'메모',
style: TextStyle(fontSize: 36, color: Colors.blue),
),
alignment: Alignment.centerLeft,
),
),
Expanded(
child: memoBuilder(context),
)
],
),
floatingActionButton: FloatingActionButton.extended(
onPressed: () {
print('[+ 메모 추가 액션버튼] 클릭');
Navigator.push(
context, MaterialPageRoute(builder: (context) => WritePage()));
},
tooltip: '+',
label: Text('메모 추가'),
icon: Icon(Icons.add),
),
);
}
Future<List<Memo>> loadMemo() async {
DBHelper dbHelper = DBHelper();
return await dbHelper.selectMemos();
}
Future<void> deleteMemo(String id) async {
DBHelper dbHelper = DBHelper();
return await dbHelper.deleteMemo(id);
}
void showAlertDialog(BuildContext context) async {
await showDialog(
context: context,
barrierDismissible: false,
builder: (BuildContext context) {
return AlertDialog(
title: Text('삭제 경고'),
content: Text('정말 삭제하시겠습니까?\n삭제된 메모는 복구되지 않습니다.'),
actions: [
FlatButton(
child: Text('삭제'),
onPressed: () {
Navigator.pop(context, '삭제');
setState(() {
deleteMemo(deleteId);
});
deleteId = '';
},
),
FlatButton(
child: Text('취소'),
onPressed: () {
deleteId = '';
Navigator.pop(context, '취소');
},
)
],
);
});
}
Widget memoBuilder(BuildContext parentContext) {
return FutureBuilder(
builder: (context, snapshot) {
if (!snapshot.hasData || snapshot.data.isEmpty) {
return Center(
child: Text(
'지금 바로 "메모 추가" 버튼을 눌러\n 새 메모를 추가해보세요!',
style: TextStyle(
fontSize: 15,
color: Colors.blueAccent,
),
textAlign: TextAlign.center,
));
} else {
return ListView.builder(
itemCount: snapshot.data.length,
itemBuilder: (context, index) {
Memo memo = snapshot.data[index];
return InkWell(
onTap: () {
print('탭 클릭');
},
onLongPress: () {
print('길게 누름');
print(memo.id);
deleteId = memo.id;
setState(() {
showAlertDialog(parentContext);
});
},
child: Container(
height: 90,
margin:
EdgeInsets.only(left: 20, top: 0, bottom: 15, right: 20),
padding:
EdgeInsets.only(left: 15, top: 10, bottom: 15, right: 15),
alignment: Alignment.center,
child: Column(
children: [
Container(
child: Text(
memo.title,
style: TextStyle(
fontSize: 20, fontWeight: FontWeight.w500),
),
alignment: Alignment.topLeft,
),
Container(
child: Text(
memo.text,
style: TextStyle(fontSize: 15),
textAlign: TextAlign.left,
),
alignment: Alignment.topLeft,
),
Container(
child: Text(
'최종 수정 시간 : ${memo.editedTime.split('.')[0]}',
style: TextStyle(fontSize: 12),
),
alignment: Alignment.bottomRight,
),
],
),
decoration: BoxDecoration(
color: Colors.white,
border: Border.all(color: Colors.blue, width: 1),
boxShadow: [BoxShadow(color: Colors.blue, blurRadius: 3)],
borderRadius: BorderRadius.circular(12),
),
),
);
},
);
}
},
future: loadMemo(),
);
}
}
반응형
'Flutter Mobile App > Flutter 메모 앱' 카테고리의 다른 글
[Flutter] 메모 11 (0) | 2020.12.28 |
---|---|
[Flutter] 메모 10 (0) | 2020.12.28 |
[Flutter] 메모 8 (0) | 2020.12.27 |
[Flutter] 메모 7 (0) | 2020.12.27 |
[Flutter] 메모 6 (0) | 2020.12.27 |